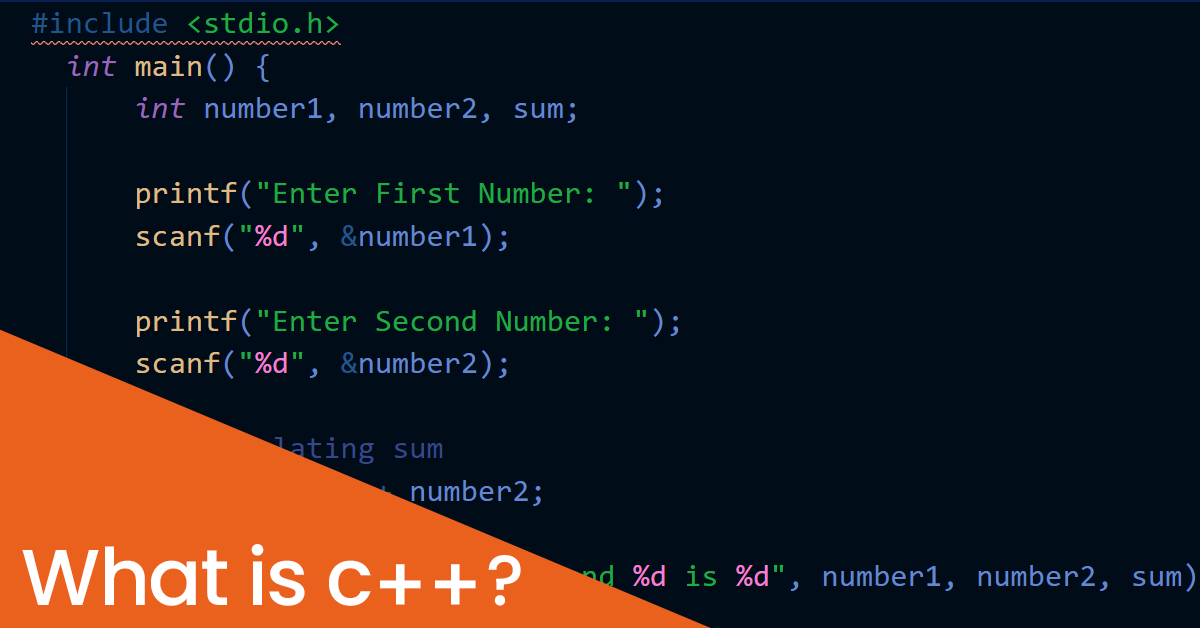
C++ is a powerful and helpful programming language. Both structured and object-oriented applications can be written in C++. Since C++ is a superset of C, most C constructs are valid in C++ and retain their meaning. There are several additions and exceptions, though.
Each group of letters separated by white space in a C++ program’s source code is referred to as a token by the compiler. The minor individual units in a program are tokens. Tokens are used while writing C++ programs. The following components are present:
- Keywords
- Identifiers
- Constants
- Strings
- Operators
Reserved words, usually referred to as keywords, are always written or typed in short (lowercase) and have specific meanings for the C++ compiler. Words like the void, int, public, etc., that the language employs for a particular function are examples of keywords. It cannot be used as the name of a variable or function. The table for the entire list of C++ keywords can be seen below.
How identifier differs from keywords and what it is:
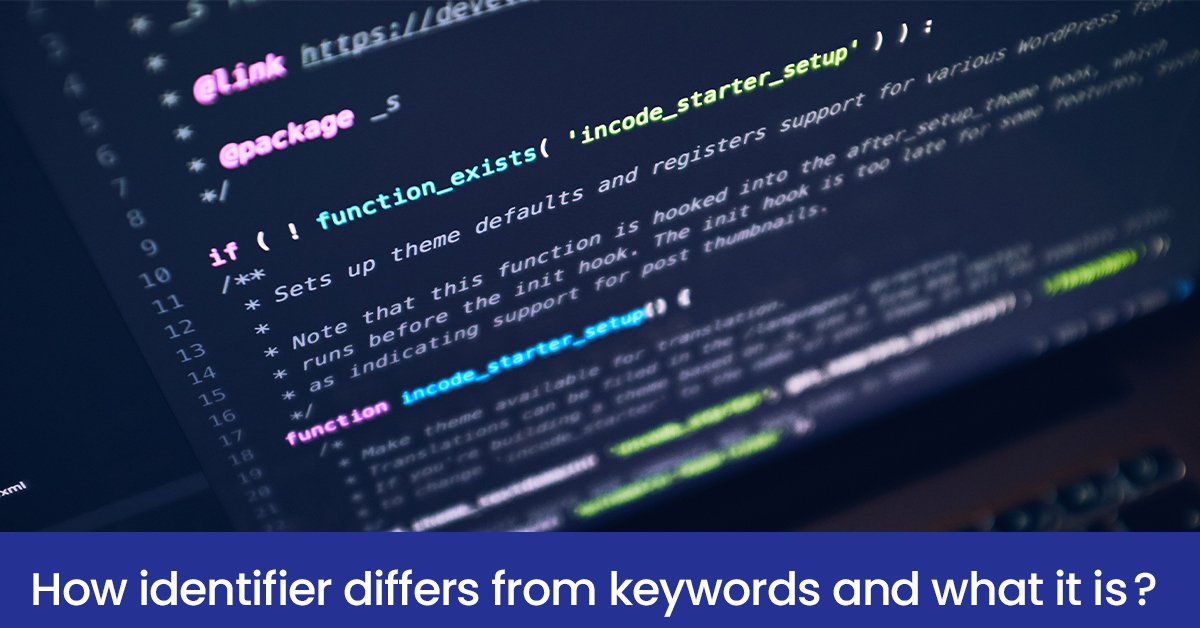
Identifiers are the names given to the programmer-created variables, functions, arrays, classes, etc. They are a necessary condition for any language.
Identifier naming conventions:
A number or other unique character cannot begin the name of the identifier.
You cannot use a keyword as an identification name.
Only numbers underscore, and alphabetic letters are allowed.
There is a distinction between capital and lowercase letters. A and an are distinct in C++, for example.
GFG, gfg, and geeks for geeks are all acceptable identities.
How are keywords distinct from IDs at this point?
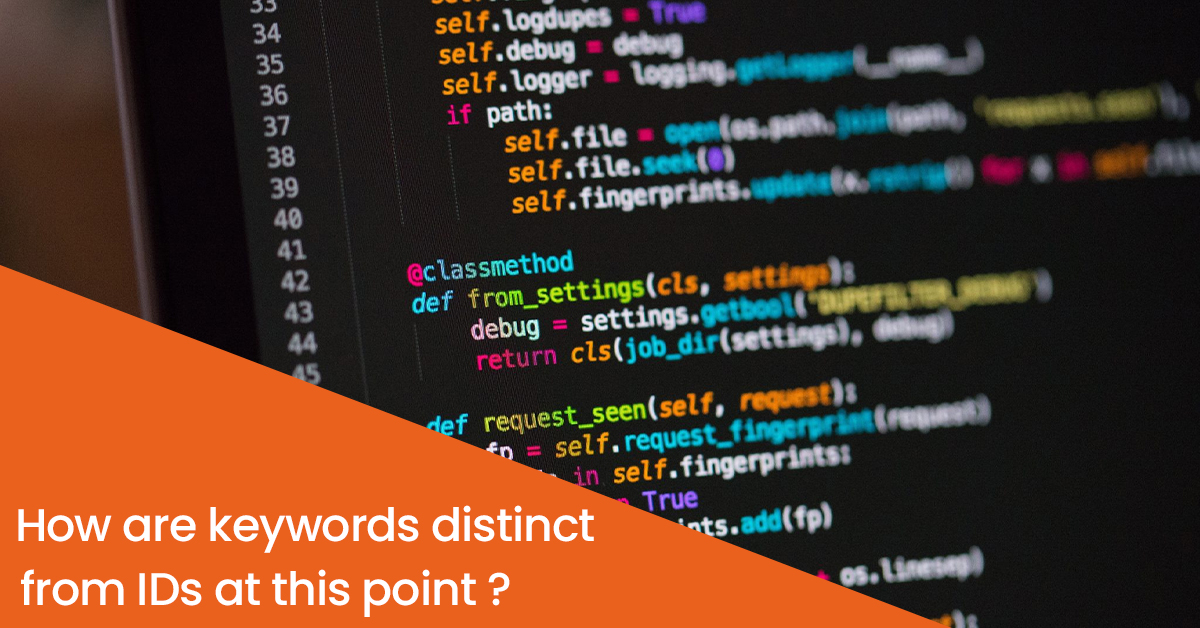
There are a few fundamental characteristics of keywords that set them apart from identifiers:
- While identifiers are the values used to construct various programming objects like variables, integers, structures, and unions, keywords are predefined or reserved words.
- While identifiers can also begin with a capital letter, keywords always start with a lowercase letter.
- Identification can include alphabetic characters, numbers, and underscores, but a keyword must only have alphabetical characters.
- Punctuation is used in keywords and identifiers; there is no particular symbol. An identification can only contain the underscore character.
Examples of IDs and keywords
- Keywords: while, do, int, char.
- Geeks-for-Geeks, GFG, and Gfg1 are identifiers.
Now that you know more about C++ Programming, here are the keywords:
- alignas
- alignof
- and
- and_eq
- asm
- atomic_cancel
- atomic_commit
- atomic_noexcept
- auto
- bitand
- bitor
- bool
- break
- case
- catch
- char
- char8_t
- char16_t
- char32_t
- class
- compl
- concept
- const
- consteval
- constexpr
- Content
- const_cast
- continue
- co_await
- co_return
- co_yield
- decltype
- default
- delete
- do
- double
- dynamic_cast
- else
- enum
- explicit
- export
- extern
- false
- float
- for
- friend
- goto
- if
- inline
- int
- long
- mutable
- namespace
- new
- noexcept
- not
- not_eq
- nullptr
- operator
- or
- or_eq
- private
- protected
- Public
- reflexpr
- register
- reinterpret_cast
- requires
- return
- short
- signed
- sizeof
- static
- static_assert
- static_cast
- struct
- switch
- synchronized
- template
- this
- thread_local
- throw
- true
- try
- typedef
- typeid
- type name
- union
- unsigned
- using
- virtual
- void
- volatile
- wchar_t
- while
- xor
- xor_eq